Webhook - Send Custom Request - Template Variables Guide
Learn how to build complex webhooks using template variables.
Prerequisites
Overview
If your custom request requires a complex payload, such as nested JSON or XML, you will use the Templates option to construct a payload in the format needed by the vendor’s endpoint. Data layer attributes can be mapped to custom Template Variables, which in turn can be referenced within any template. This guide will show how to create Template Variables for both single key/value pairs and for array values.
Single Value Variables
The most common use for a Template Variable is to pass dynamic values from an Attribute into a placeholder within a Template. A Template Variable can be referenced in any Template by surrounding its name in double curly braces e.g. {{VARIABLE NAME}}
.
Here is an example payload required for tracking the status of a Shopping Cart:
{
"userEmail" : "bob@email.com",
"total" : 9.99,
"discount" : "10%",
"date" : "2016-07-13T12:30:59.000Z"
}
You can see that each value will be dynamic and must be populated by a corresponding Attribute. The names for each value in this payload are the vendor’s requirements for the format of the request. Template Variables are how you populate Attribute values into a request like this.
The Template required to create this payload might look like this, where the values have been replaced by placeholders that reference the Template Variables we are going to use:
{
"userEmail" : "{{userEmail}}",
"total" : {{itemTotal}},
"date" : "{{date}}",
"discount" : "{{discount}}"
}
Here is how you can accomplish this using Template Variables:
- Start by identifying the Attributes you wish to include in the Body Data. For this example, you will use the following Cart details:
Data Layer Attribute Type Template Variable Value Cart User Email String userEmail
user@example.com
Cart Total Number itemTotal
9.99
Cart Date Date date
2016-07-13T12:30:59.000Z
Cart Discount String discount
10%
- In the Template Variables section, map each Attribute to a corresponding Template Variable name of your choosing.
- In the Templates section, construct the appropriate template according to the vendor’s specifications. Be sure to give it a useful name since this will also be referenced with double curly braces later on. We called this template “cartdetails”.
- In the Body Data section, map the Custom Value of
{{cartdetails}}
to the Body.
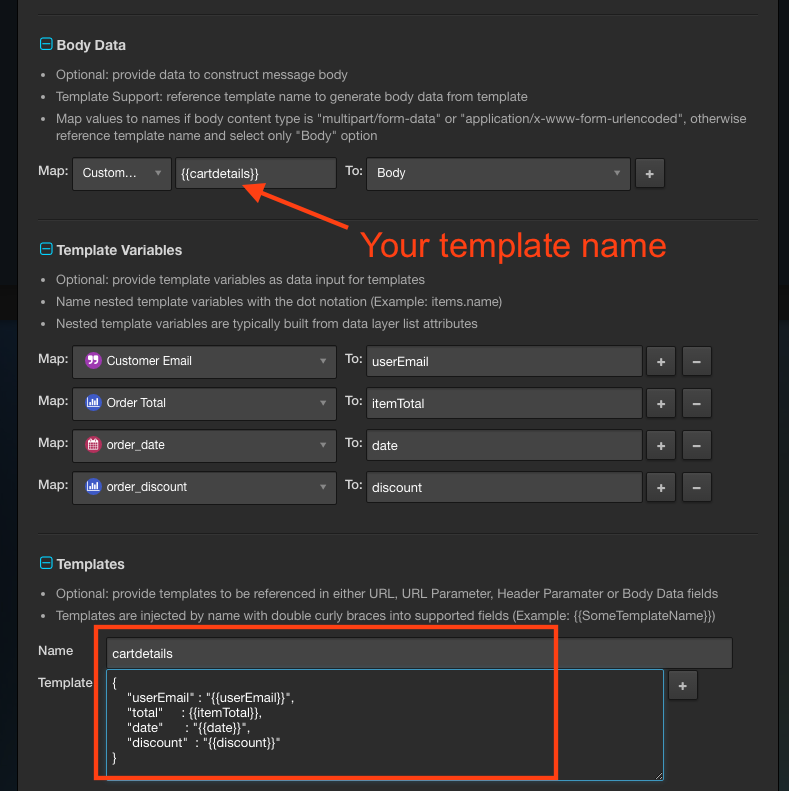
Nested Map: One Level Array of Objects
To construct a JSON array of objects, you must use an Array Attribute and the special “dotted names” convention in the naming of your Template Variables. When using this notation, the part of the name coming before the “.” will automatically enable the use of a section within the Template referred to by that name. Let’s look at an example to see how this works.
Here is an example payload required for tracking Shopping Cart content:
{
"cart": [{
"item" : "Plate",
"quantity": "1",
"price" : "5.00",
"id" : "001"
},
{
"item" : "Fork",
"quantity": "2",
"price" : "0.99",
"id" : "002"
},
{
"item" : "Mug",
"quantity": "1",
"price" : "6.99",
"id" : "003"
}]
}
In order to construct a payload like that we would need a Template like this:
{
"cart": [
{{#cart}}
{
"item" : "{{item}}",
"quantity" : "{{quantity}}",
"price" : "{{price}}",
"id" : "{{id}}"
}{{#iter.hasNext}}, {{/iter.hasNext}}
{{/cart}}
]
}
Using this example, you would use cart as the prefix in your Template Variables using dotted names e.g. cart.item
Here we can see how the necessary Template Variables would be defined:
Data Layer Attribute | Type | Template Variable | Value |
---|---|---|---|
Cart Items | Array of Strings | cart.item |
["Plate", "Fork", "Mug"] |
Cart Quantities | Array of Numbers | cart.quantity |
["1", "2", "1"] |
Cart Prices | Array of Numbers | cart.price |
["5.00", "0.99", "6.99"] |
Cart Item IDs | Array of Strings | cart.id |
["001", "002", "003"] |
Limitations for array of objects
- Only one level nested elements are supported. Deeper JSON structures are not possible.
- Data Layer Attributes must all be arrays of identical length.
Cascade Map: Set Value Across Objects
It is possible to cascade a single data attribute across every array object. All non-set-of-string attributes are cascaded as long as the variable name follows the special “period” character notation.
Example:
Data Layer Attribute | Attribute Type | Variable | Value |
---|---|---|---|
Cart Items | Set of Strings | cart.item |
[ "Plate", "Fork", "Mug" ] |
Transaction ID | String | cart.transactionId |
T1284 |
Resulting JSON:
{
"cart": [
{
"item": "Plate",
"transactionId": "T1284"
},
{
"item": "Fork",
"transactionId": "T1284"
},
{
"item": "Mug",
"transactionId": "T1284"
}
]
}
This page was last updated: February 21, 2023