Consent Management
Learn to implement Consent Management for iOS (Objective-C).
Usage
Usage of this module is recommended, as it is automatically included and enabled in the CocoaPods framework builds.
Supported platforms include: iOS, macOS, tvOS, and watchOS.
For more information about Consent Management, see Consent Management.
Sample Apps
To help familiarize yourself with enabling Tealium Consent Management, it is recommended to download the sample apps:
Data Layer
The following variables are transmitted with each tracking call while Consent Management is enabled:
Variable Name | Description | Example |
---|---|---|
policy |
The policy under which consent was collected (Default: gdpr ) |
gdpr |
consent_status |
The user’s current consent status | ["Consented" , "Not Consented" ] |
consent_categories |
The user’s current categories they are consented to | @[@"analytics"] |
Use Cases
Use Case: Simple Opt-in
[_tealium.consentManager setUserConsentStatus:Consented];
This example shows you how to give your users a simple “Opt-in/Opt-out” option. If the user consents, they are automatically opted-in to all tracking categories. If they revoke their consent, no categories are active, and no tracking calls are sent.
Define and call the following method in your Tealium Helper class when your app user consents to or declines tracking. If the user consents to tracking, the Consent Manager automatically opts them in to all tracking categories.
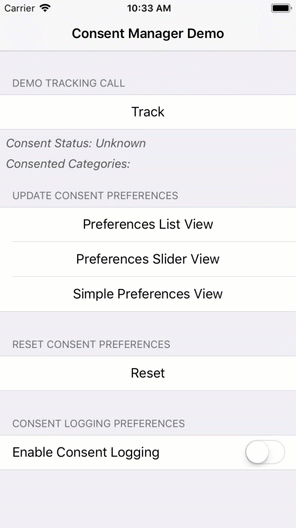
Consent Simple Opt-In
Use Case: Grouped Opt-in
[_tealium.consentManager setUserConsentStatus:Consented withUserConsentCategories:@[@"analytics", @"monitoring", @"big_data", @"mobile", @"crm"]];
This example shows a category-based consent model, where tracking categories are grouped into a smaller number of higher-level categories, defined by you. For example, you may choose to group the Tealium consent categories "analytics"
, "monitoring"
, "big_data"
, "mobile"
, and "crm"
under a single category called "performance"
. This may be easier for the user than selecting from the full list of categories. You may choose to represent this in a slider interface, ranging from least-permissive to most-permissive (all categories).
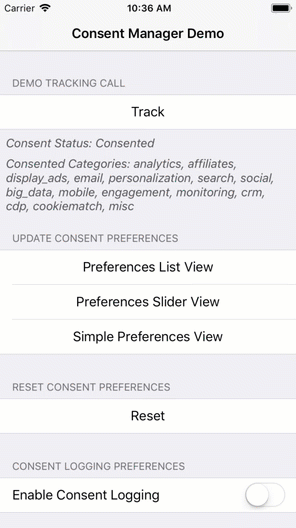
Consent Group Opt-In
Use Case: Category-based Opt-in
[_tealium.consentManager setUserConsentStatus:Consented withUserConsentCategories:@[@"analytics", @"personalization"]];
This example shows a category-based consent model where the user must explicitly select each category from the full list of categories. The default state of the Consent Manager is "Unknown"
, which queues hits until the user provides their consent. If the user consents to any category, events are dequeued, and the consented category data is appended to each tracking call.
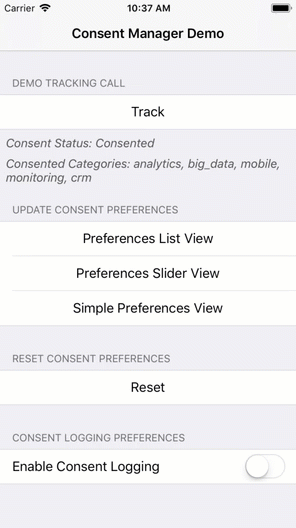
Consent Category Opt-in
API Reference
consentManager
This code shows how to enable Consent Management on Tealium initialization.
// TealiumHelper.h
@import TealiumIOS;
@interface TealiumHelper : NSObject<TealiumDelegate, TEALConsentManagerDelegate>
@property (nonatomic, strong) Tealium *tealium;
@end
// TealiumHelper.m
@implementation TealiumHelper
+ (instancetype _Nonnull)sharedInstance {
static TealiumHelper *tealiumHelper = nil;
static dispatch_once_t onceToken;
dispatch_once(&onceToken, ^{
tealiumHelper = [[self alloc] init];
});
return tealiumHelper;
}
- (instancetype)init {
if (self = [super init]) {
TEALConfiguration *configuration = [TEALConfiguration configurationWithAccount:@"ACCOUNT" profile:@"PROFILE" environment:@"ENVIRONMENT"];
[configuration setLogLevel: TEALLogLevelDev];
// enable Tealium Consent Manager
[configuration setEnableConsentManager:YES];
_tealium = [Tealium newInstanceForKey:@"INSTANCE" configuration:configuration];
_tealium.delegate = self;
// set optional Tealium Consent Manager Delegate
_tealium.consentManagerDelegate = self;
NSLog(@"%s, %@", __FUNCTION__, [TEALConsentManager consentStatusString:_tealium.consentManager.userConsentStatus]);
}
return self;
}
@end
enable
Enable Consent Manager. This method enables Consent Manager at a later time and not on Tealium initialization.
[_tealium.consentManager enable];
The recommended approach is to enable Consent Manager on Tealium initialization.
isEnabled
Checks if Consent Manager is enabled.
[_tealium.consentManager isEnabled];
disable
Disables Consent Manager. This method disables Consent Manager after Tealium is fully initialized.
[_tealium.consentManager disable];
isDisabled
Checks if the Consent Manager is disabled.
[_tealium.consentManager isDisabled];
consentManagerDelegate
Registers a specific class conforming to the TEALConsentManagerDelegate protocol.
[_tealium setConsentManagerDelegate:(id<TEALConsentManagerDelegate>)];
setUserConsentStatus
Sets the user’s consent status. Designed to be called from your consent management preferences screen in your app when the user enables or disables tracking.
[_tealium.consentManager setUserConsentStatus:Consented];
Consent Manager always sets the list of consented categories to include ALL available consent categories if the status is Consented
. This method does not allow categories to be set selectively.
Parameters | Description | Example |
---|---|---|
TEALConsentStatus |
A value from TEALConsentStatus enum | [Consented , NotConsented , Disabled ] |
setUserConsentCategories
Sets the user’s consent categories. Designed to be called from your consent management preferences screen in your app.
[_tealium.consentManager setUserConsentCategories:@[@"analytics"]];
Always sets consent status to Consented
when any number of categories are set.
Parameters | Description | Example |
---|---|---|
NSArray |
An array of predefined NSString categories | @[@"analytics", @"big_data"] |
setUserConsentStatus:withUserConsentCategories
Sets the user’s consent status and user’s consent categories in a single method.
[_tealium.consentManager setUserConsentStatus:Consented withUserConsentCategories:@[@"analytics"]];
Parameters | Description | Example Value |
---|---|---|
TEALConsentStatus | A value from TEALConsentStatus enum | [Consented , NotConsented ] |
NSArray | An array of predefined NSString categories | @[@"analytics", @"big_data"] |
userConsentStatus
Returns the current consent status of the user.
[_tealium.consentManager userConsentStatus];
userConsentCategories
Returns the current consent categories of the user.
[_tealium.consentManager userConsentCategories];
isConsented
Convenience method to determine if the user is consented.
[_tealium.consentManager isConsented];
consentStatusString
Convenience method for human-readable string of the TEALConsentStatus enum.
[TEALConsentManager consentStatusString:_tealium.consentManager.userConsentStatus];
resetUserConsentPreferences
Resets all currently stored consent preferences. Reverts to Unknown
consent status with no categories.
[_tealium.consentManager resetUserConsentPreferences];
setConsentLoggingEnabled
Enables the Consent Logging feature, which sends all consent status changes to Tealium Customer Data Hub for auditing purposes.
[_tealium.consentManager setConsentLoggingEnabled:YES];
Parameters | Description | Example Value |
---|---|---|
TEALConsentLoggingEnabled |
Enables or disables consent logging | [YES , NO ] |
isConsentLoggingEnabled
Returns the current state of the consent logging feature.
[_tealium.consentManager isConsentLoggingEnabled];
allCategories
Returns the complete list of supported consent categories.
[_tealium.consentManager allCategories];
TEALConfiguration
enableConsentManager
Sets the initial consent status for the user when the library starts up for the first time. If there are saved preferences, they override any preferences passed in the config object.
self.configuration.enableConsentManager = YES;
Consent Manager always sets the list of consented categories to include ALL available consent categories, if the status is Consented
. This method does not allow categories to be set selectively.
userConsentStatus
Sets the user’s initial consent status when the library starts up for the first time. If there are saved preferences, these override any preferences passed in the config object.
self.configuration.userConsentStatus = Consented;
Parameters | Description | Example Value |
---|---|---|
TEALConsentStatus |
A value from TEALConsentStatus enum | [Consented , NotConsented , Disabled ] |
userConsentCategories
Sets the user’s initial consent categories when the library starts up for the first time. If there are saved preferences, these override any preferences passed in the config object.
self.configuration.userConsentCategories = @[@"analytics", @"retargeting"];
Parameters | Description | Example Value |
---|---|---|
NSArray |
An array of predefined NSString categories | @[@"analytics", @"big_data"] |
TEALConsentManagerDelegate
consentManagerDidChangeWithState
This method is called whenever the Consent Manager user consent status is changed. For example, the user consented or declined tracking.
- (void)consentManagerDidChangeWithState:(TEALConsentStatus)consentStatus;
consentManagerDidUpdateConsentCategories
This method is called whenever the Consent Manager user consent categories are updated.
-(void)consentManagerDidUpdateConsentCategories:(NSArray * \_Nullable)categories;
consentManagerWillDropDispatch
This method is called whenever the Consent Manager is going to drop a tracking call. For example, the user declined tracking.
- (void)consentManagerWillDropDispatch:(TEALDispatch * \_Nullable)dispatch;
consentManagerWillQueueDispatch
This method is called whenever the Consent Manager is in the Unknown
state. Tracking calls are being queued locally until the user grants or declines consent.
- (void)consentManagerWillQueueDispatch:(TEALDispatch * \_Nullable)dispatch;
Consent Categories
The following lists all the supported consent categories:
analytics
affiliates
display_ads
email
personalization
search
social
big_data
mobile
engagement
monitoring
cdm
cdp
cookiematch
misc
TEALConsentStatus
Unknown
The Unknown
status is the default setting for the Consent Manager. In this state, the Consent Manager queues events locally until an affirmative Consented
/ NotConsented
status is provided.
self.tealium.consentManager.userConsentStatus = Unknown
Consented
The Consented
status is set when the user has consented to tracking. In this state, the Consent Manager allows all tracking calls to continue as normal.
self.tealium.consentManager.userConsentStatus = Consented
NotConsented
The NotConsented
status is set when the user has declined tracking. In this state, the Consent Manager drops all tracking calls and halt further processing by the SDK.
self.tealium.consentManager.userConsentStatus = NotConsented
This page was last updated: April 9, 2024